4 Nutrients distribution
This section presents the analyses that illustrates the distribution of nutrients within various components of small-scale fisheries in East Timor.
4.1 Fish groups
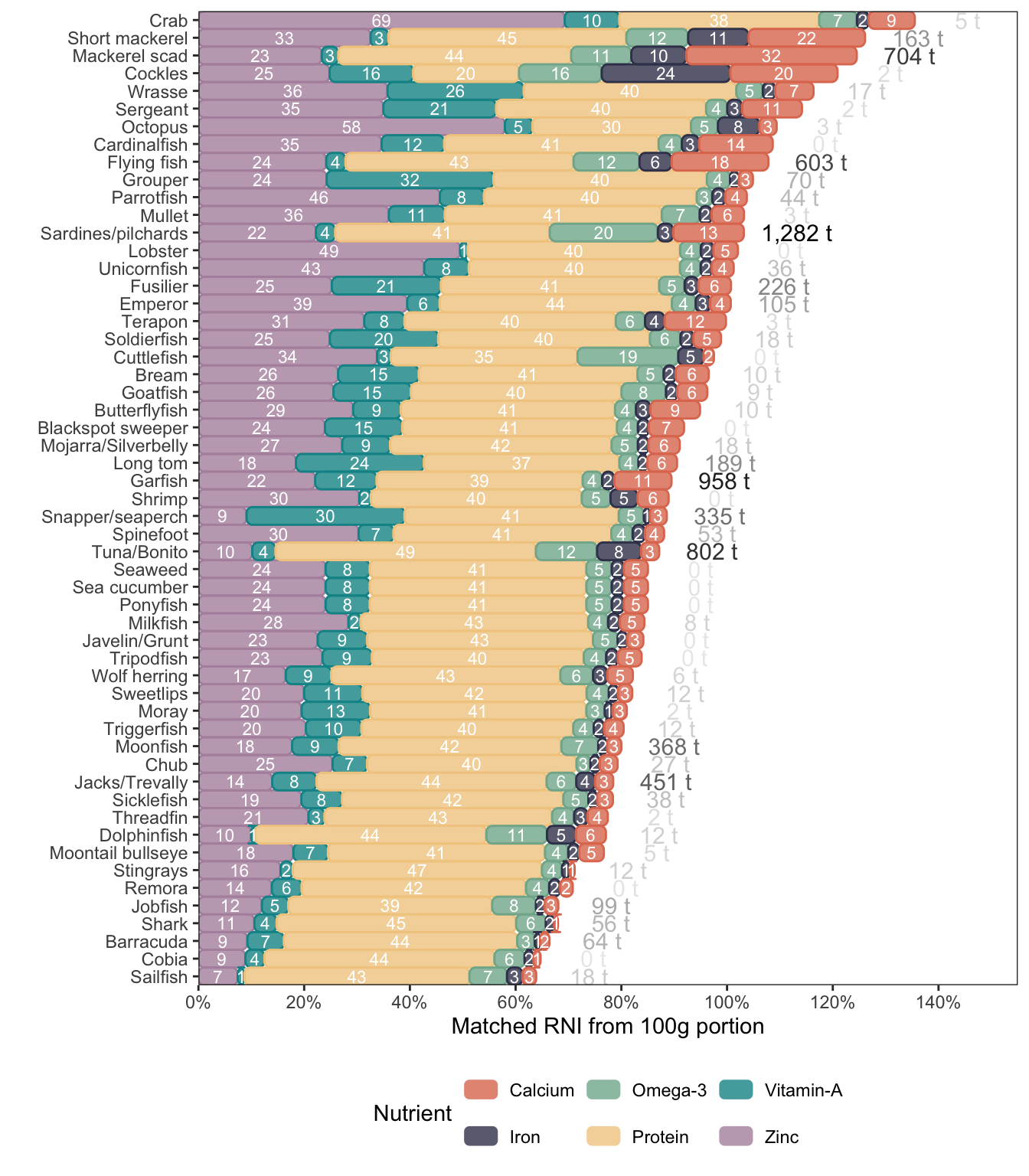
Figure 4.1: The bar chart illustrates the contribution of a variety of marine food sources to the Recommended Nutrient Intake (RNI) for six fundamental nutrients, based on a 100g portion. Each bar is a color-segmented stacked visual, with distinct hues corresponding to individual nutrients, and white numbers within indicating the specific percentage contribution of each nutrient. The chart incorporates the mean annual catch in metric tons for each marine species from 2018 to 2023, presented at the end of each bar, providing a view of both the nutritional value and the harvest volume of these essential food sources. The transparency of these values is adjusted to reflect each species’ relative contribution to the mean annual catch
4.2 Habitat and gear type
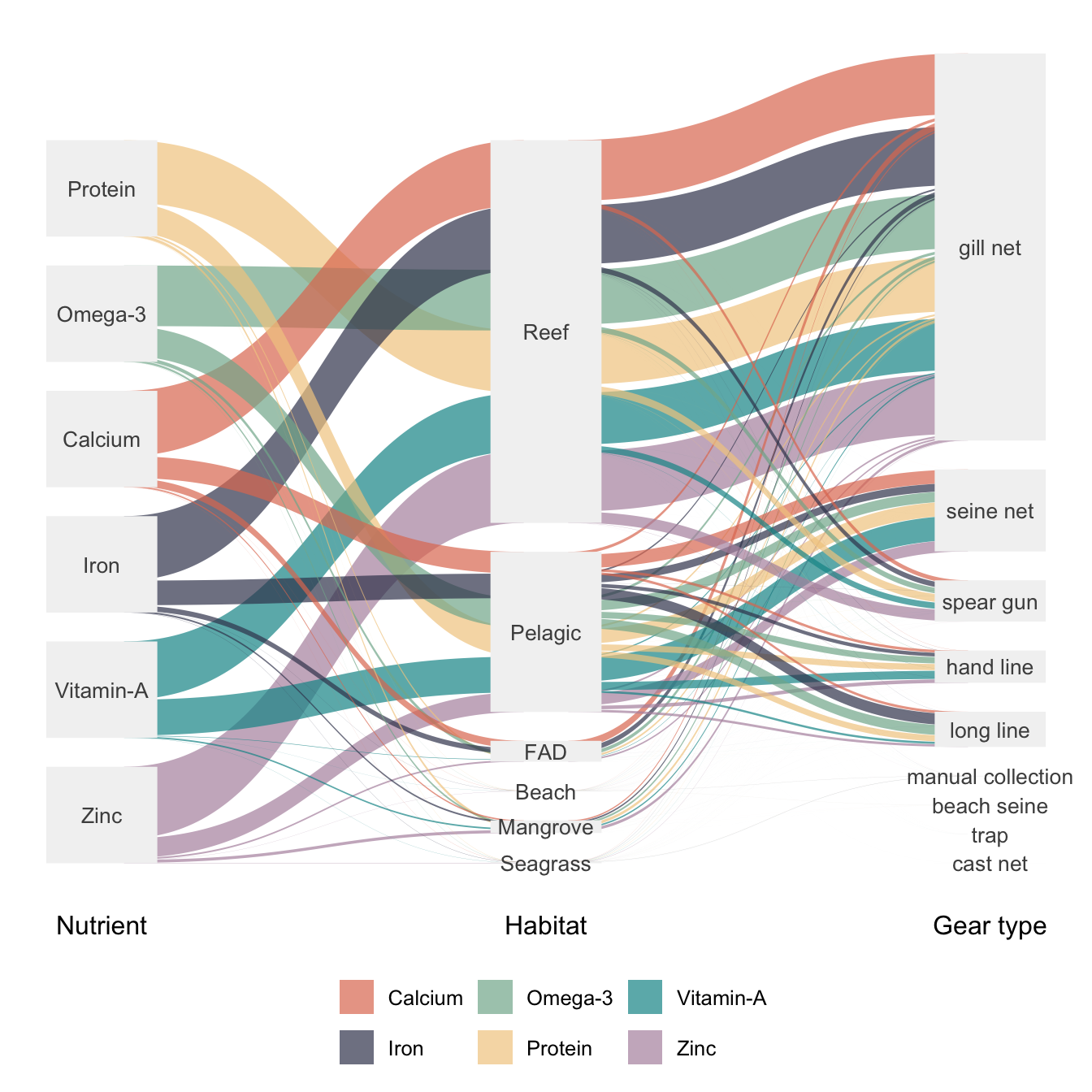
Figure 4.2: Sankey diagram showing the relative distribution of key nutrients across various marine habitats and the corresponding extraction by different fishing gear types used in Timor-Est small-scale fisheries.
4.3 Nutritional contribution and economic profiling
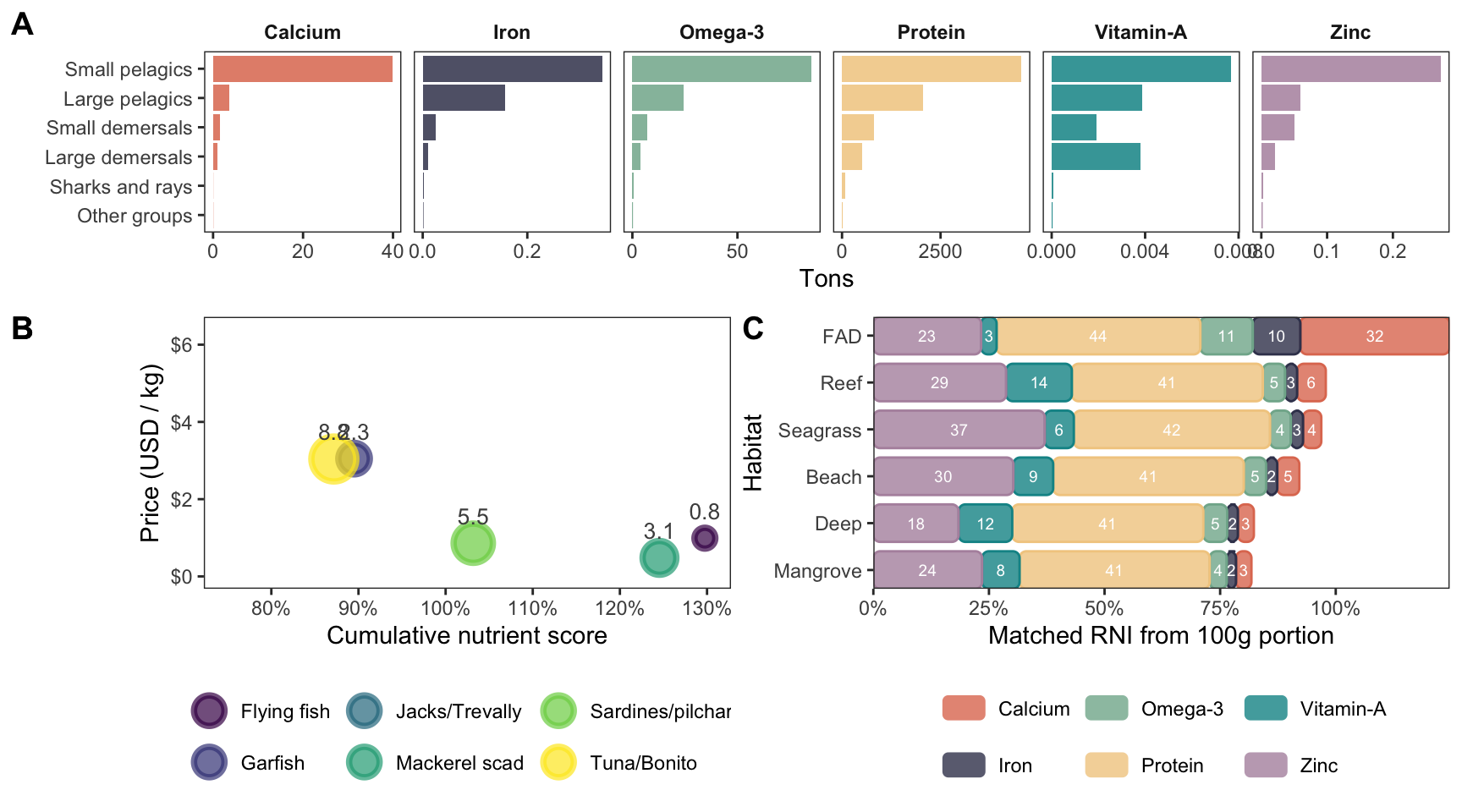
Figure 4.3: Nutritional and economic profiling of key fish groups within the Timor-Leste fishery.Panel A, Distribution of nutritional content among different functional fish groups: Small pelagics, Large pelagics, Small demersals, Large demersals, Sharks and rays and Other groups, that includes shrimps, molluscs, cephalopods and crustaceans. The plot shows the ranked contribution of each functional fish to the supply of calcium, omega-3, iron, protein, vitamin A, and zinc during the period 2018-2023. Panel B, Comparative analysis of nutritional score versus economic accessibility for key fish groups. This scatter plot displays the relationship between the cumulative nutritional score and the market price for various fish groups within Timor-Leste fishery. The x-axis quantifies the cumulative contribution to the Recommended Nutrient Intake (RNI) for six essential nutrients (zinc, protein, omega-3, calcium, iron, vitamin A) from a 100g portion of each fish group. The y-axis represents the average market price per kilogram for each group. Dot size and the accompanying numerical labels reflect the relative catch percentage of each group, serving as an index of accessibility and availability. Panel C, The bar chart illustrates the contribution of each habitat to the Recommended Nutrient Intake (RNI) for six fundamental nutrients, based on a 100g portion. Each bar is a color-segmented stacked visual, with distinct hues corresponding to individual nutrients, and white numbers within indicating the specific percentage contribution of each nutrient.